How to add JavaScript to HTML is the last of a 3-parts series on how to make a simple website. JavaScript is a high-level language used to create web-based applications. It is one of the 3 core web technologies used in web designing and development.
JavaScript is the third and the last of the 3 core web technologies we will discuss in this series. Unlike the HTML that creates structure, and the CSS used for layouts; JavaScript is used for interactivity.
A website is made up of structure, layout, and human interaction. HTML is the foundational technology that creates the website’s structure. While the layout and aesthetics of the website are handled by the CSS. Although HTML has some elements that are used to create user interactions, JavaScript (JS) is designed for this purpose.
Some of the HTML5 elements that allow for users’ interactions include the <canvas>, <audio>, <form>, and <video> elements. Outside these elements, there is no such element that can be used to create web application.
To create web applications such as a Calculator or a Game, we need a high-level programming language. Because modern web browsers have built-in engines that support JavaScript, JS becomes the preferred language for web development.
We usually insert JavaScript codes in an HTML file which is a markup language. In this article, you will learn how to add JavaScript to HTML.
To follow up with this tutorial, you will need the previous two tutorials or download the codes:
Download previous codes and continue working from there using the following links:
- How to make HTML websites (download codes from Github)
- How to add CSS to HTML (download codes from Github)
You will learn the following:
- How to add JavaScript to HTML
- Conclusion
What is JavaScript?
JavaScript is a high-level programming language used to create human interaction in web designing and development. Using JS, we can create different kinds of web apps that enhance user experience.
First developed in 1995 by a Netscape employee (Brandan Eich), JS is widely used today and is widely supported. It is also known as ECMAScript with versions named ES1 – ES7; also, written as ECMAScript 2016, etc.
In web development, JS can be used in the front-end and backend. Some JavaScript libraries and frameworks can help speed up web development. Examples include Electron, React, Vue, Angular, Svelte, etc.
Introduction to JavaScript
JavaScript codes are placed in the HTML files and rendered on the browser. This is why we are learning how to add JavaScript to HTML. To do this, we introduced tools and platforms to be used as follow:
- Text editor & IDEs (Bracket, NetBean, Atom, Notepad++, VSCode, etc)
- Platforms (JSFiddle, Liveweave, PlayCode, Github, etc.)
In this practice, we will use Sublime Text editor and the Chrome browser.
JS syntax
A syntax in JS defines how a line of code in JS is written. There are sets of rules that guidelines of code are written in every programming language. The same applies to JS. For example, in JavaScript, each line of code ends with a semicolon (;).
Let us illustrate:
<script type="text/javascript"> //Simple example of javascript syntax const taxPerc = 0.075; var productAmt = 3500; let amtDue = productAmt*taxPerc ; console.log(amtDue); </script>
Notice the following from the above code:
- The line that starts with // is the comment line. Every code on this line will not be executed. You can create a multiline comment by using /* place more than one line comment here */
- Each line of code ends with a ;
- Each line of code began with any of const, var, or let. Learn the differences between const, var, and let here.
- The variable names were written in camelCase.
This is a typical way to write JavaScript syntax.
Notice the code: console.log(amtDue).
This code is used to display the result in the browser console. To access the console, press F12 when on the browser window.
JavaScript is case-sensitive. This means that amtdue is not the same as amtDue. Please, avoid making such mistakes when writing codes.
Variable and assignments
Variables may be defined as a container that holds values. There are three ways to declare a variable in JavaScript namely const, var, and let. Each of these keywords is used for different purposes. For example, the const keyword is used for a value that cannot change, e.g., pi. var and let differ in scope when used, but their value can change.
var can be accessed throughout the scope of a function while let can be accessed within a block. Therefore, while writing a function, you can use let if you want a variable to terminate within a block. If you want a function wide variable, use the var keyword.
Hence, a variable declaration is done by simply using any of the three keywords followed by a variable name. You can declare a variable as follow:
var apple, mango, total; const fees; let sum, subTotal;
Assigning a value to a variable is called assignment. This is done using the ‘=’ (equal sign). A variable can be declared and value assigned at the same time or later. For example;
<script type="text/javascript"> let subTotal, vat, totalAmt; const taxPercent = 0.075; var productQty = 13; var productPrice = 25; subTotal = productQty * productPrice; vat = subTotal*taxPercent; totalAmt = subTotal+vat;
From the above code, you will notice the following:
- A variable can be declared without an assignment statement
- Value can be assigned to a variable later in the code
- A variable can be declared together with an assignment statement
- A variable can be assigned another variable
Data types
A variable can be assigned different values. These values are classified as data types. There are six (6) primitive data types used in JavaScript. Objects and arrays can be used to store more than one value. Let’s look at the primitive data types.
- Number: this data type is used for integers (e.g. 25) and floating-point numbers (e.g. 15.75). Mathematical operators (- + / *, etc) can be used on such data types. Values such as Infinity and NaN also belong to this data type.
- BigInt: This data type is used to represent integer values larger than (253 – 1), or less than (-253 – 1). It is used to represent integers of arbitrary length. N is usually attached at the end of the bigInt data type.
- String: Is any type of value enclosed in a single or double quotation.
- Boolean: This is a data type that has only two values: true and false. It is used to store yes or no values. Boolean values are used when making comparisons, e.g. is A > B?
- Null: the null data type is used to store the value of nothing. When assigned to a variable, it means the variable has nothing in it.
- Undefined: the undefined data type as used in JavaScript means that value is not assigned. When you declare a variable without assigning a value to it, JS automatically assigns undefined to it.
Operators in JS
We will look at three (3) types of operators in JavaScript. They are the typeof, mathematical and conditional operators.
typeof operator
The typeof operator or keyword is used to determine the type of an argument. When you want to know the data type of a particular argument, you simply use
typeof argument |
This will return the type of argument as a number, string, etc.
Mathematical operators
These types of operators are used to perform mathematical calculations in JS. Some of the mathematical operators used in JS are tabulated below.
S/N | Operator | Usage |
1. | + | Addition of two numbers |
2. | – | Subtraction of two numbers |
3. | * | Multiplication of two numbers |
4. | / | Divides left number by right number |
5. | ** | Exponential – raises number by the exponent |
6. | ++ | Incremental – increments a number |
7. | — | Decremental – decreases a number |
8. | % | Modulus – returns the remainder after division |
Conditional and comparison operators
Conditional operators are important, especially, when making comparisons. When a logical statement is made, there is a need to determine if the statement is true or false. In this case, the conditional and comparison operators are required. They are tabulated below.
S/N | Operator | Meaning |
1. | == | Loose equal to |
2. | === | Strict equal to (equals value & data type) |
3. | != | Loose not equal to |
4. | !== | Strict not equal to (value & data type) |
5. | > | Greater than |
6. | >= | Greater than or equal to |
7. | < | Less than |
8. | <= | Less than or equal to |
S/N | Operator | Meaning |
1. | && | And operator (A and B must be true) |
2. | || | OR operator (A or B can be true) |
3. | ! | Not operator (Not A==B) |
Operations on DOM
Adding JavaScript to HTML sometimes requires that the DOM be manipulated in some ways. Remember that DOM means Document Object Model, see how to add CSS to HTML. The DOM represents HTML elements in a tree and each branch or node is an object. Each node can be manipulated using JS.
Manipulating the DOM
To manipulate the DOM using JS, you will first have access to it. In order to access the DOM in JavaScript, we use the document object with the following functions:
- getElementById()
- getElementByTagName()
- getElementByClassName()
Therefore, by adding document.getElementById(‘x’), or getElementByTagName(), etc, we can access the DOM.
Remember that in CSS, we can select elements by tag name, id, or classname. To replicate the same, we use the 3 functions above.
- To getElementById means to select the element that has this specific id.
- To getElementByTagName() means to select all the elements that has this tag
- To getElementByClassName() means to select all the elements that have this class name.
Let us illustrate with this code.
<h3>Simple Examples:</h3> <div id="result"> <p id="subt"></p> <p id="tax"></p> <p id="total"></p> </div> <script type="text/javascript"> let subTotal, vat, totalAmt; const taxPercent = 0.075; var productQty = 13; var productPrice = 25; subTotal = productQty * productPrice; vat = subTotal*taxPercent; totalAmt = subTotal+vat; document.getElementById('subt').innerHTML= "Subtotal: " + subTotal; document.getElementById('tax').innerHTML= "VAT: " + vat; document.getElementById('total').innerHTML= "Total Amount: " + totalAmt; </script>
How to add JavaScript to HTML
How to add JavaScript to HTML is as simple as adding CSS to HTML. There are three (3) methods:
- The inline method
- The embedded or internal method, and
- The external method
If you have learned how to add CSS to HTML, you’ve almost known how to add JavaScript to HTML.
Add inline JavaScript to HTML
To add inline JavaScript to HTML, you will add your code in the opening tag of the HTML element.
Let us illustrate with this example:
<h1>JS Examples</h1> <div id="ex"> </div> <br> <button onclick="document.getElementById('ex').innerHTML=Date();"> Click to Show Date </button>
Run the above code in a browser and click on the button to display the current system date and time.
Add internal JavaScript to HTML
At this time, the code in the opening tag will be removed and taken to the head section. Best practices advise that the code be placed before the </body> closing tag for a better user experience. Whichever place the code is placed, you will get the same result.
For example, in the code above will be rewritten thus;
<!doctype html> <html> <head> <title> Examples</title> </head> <body> <!—HTML version of the code as it will be displayed in the browser--> <h1>JS Examples</h1> <div id="ex"> </div> <br> <button onclick="ShowDate();"> Click to Show Date </button> <!—Javascript code that will be executed when button is clicked--> <script type="text/javascript"> function ShowDate(){ document.getElementById('ex').innerHTML=Date(); } </script> </body> </html>
The main code is created as a function that will run when the button is clicked. On the opening tag, the function will be called onclick.
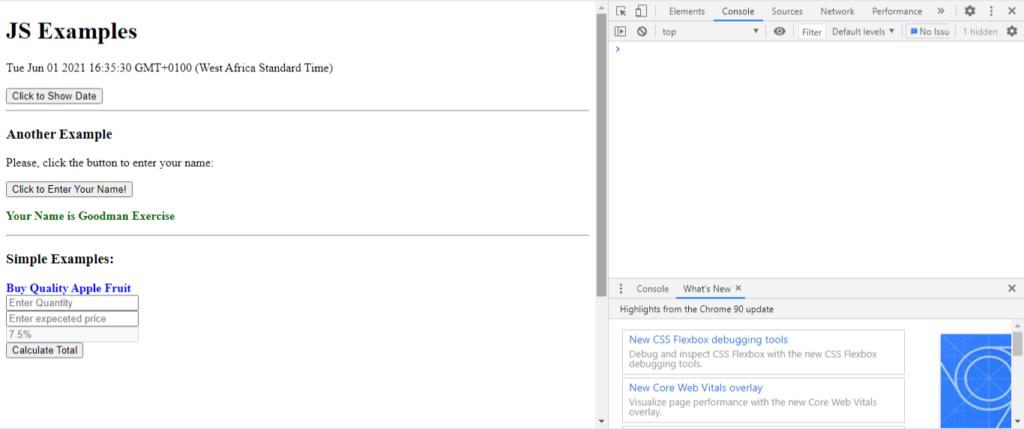
Add external JavaScript to HTML
External JavaScript works like external HTML. In this case, all the JS codes are stored in an external file with the .js extension. The file is then included in the HTML file. You can decide to include the file in the <head> section or before the closing </body> tag.
The following code illustrates the possibility.
HTML
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>My JS Examples</title> </head> <body> <h1>JS Examples</h1> <div id="ex"> </div> <br> <button onclick="ShowDate();"> Click to Show Date </button> <hr> <h3>Another Example</h3> <p>Please, click the button to enter your name:</p> <button onclick="EnterText();"> Click to Enter Your Name! </button> <p id="name" style="font-weight:900; color: darkgreen;"> </p> <!--This is used for simple example--> <h3>Simple Examples:</h3> <div id="result"> <p id="subt"></p> <p id="tax"></p> <p id="total"></p> </div> <!--This is used for simple calculator example--> <h3>Simple Calculator Example:</h3> <form> <fieldset style="width: 20%;"> <legend style="font-weight: 900; color: blue;">Buy Quality Apple</legend> <div> <label>Quantity</label><br> <input id="qty" type="number" name="tax" placeholder="Enter Quantity"> </div> <div> <label>Price</label><br> <input id="pri" type="number" name="price" placeholder="Enter expected price"> </div> <div> <label>Tax%</label><br> <input id="taxv" type="number" name="tax" disabled="" placeholder="7.5%" value="0.075"> </div><br> </fieldset> </form> <button style="width: 20%; margin-left: 20px" onclick="CalculateCost();">Calculate Cost!</button> <hr> <div id="result" style="background: #cccccc; display: none;"> <table cellpadding="2"> <tr> <th colspan="2">Total Cost of Products Bought</th> </tr> <tr> <td>Sub Total:</td> <td id="subt"></td> </tr> <tr> <td>Vat (Tax):</td> <td id="tax"></td> </tr> <tr> <td>Total Amount:</td> <td id="total"></td> </tr> </table> </div> <script type="text/javascript" src="script.js"></script> </body> </html>
JavaScript Code:
//This code is used to show the date and time of the day function ShowDate(){ document.getElementById('ex').innerHTML=Date(); } //This code is used to allow users to enter their name at the system prompt function EnterText(){ var txt; var txtEntered = window.prompt("Enter Your Full Name"); if(txtEntered != null || txtEntered != ""){ txt = "Your Name is " + txtEntered; } document.getElementById('name').innerHTML = txt; } //Codes to calculate the cost of the product purchased function CalculateCost(){ let subTotal, vat, totalAmt; const taxPercent = document.getElementById('taxv'); var productQty =document.getElementById('qty'); var productPrice = document.getElementById('pri'); subTotal = productQty.value * productPrice.value; vat = subTotal * taxPercent.value; totalAmt = subTotal + vat; document.getElementById('result').style.display = ""; document.getElementById('subt').innerHTML= subTotal; document.getElementById('tax').innerHTML= vat; document.getElementById('total').innerHTML= totalAmt; }
The three methods illustrate how to add JavaScript to HTML. But the third method is the recommended method.
Conclusion
JavaScript is an impressive web application programming language. Though learning how to use it is tasking, it is a must-learn for web designers. Therefore, if you are desiring to learn web designing, you must get started learning HTML, CSS, and JavaScript.
How to add JavaScript to HTML is simple and straightforward. Learning any single method can do, but to create a functional web app, the external method is ideal.
This article introduced you to JavaScript and how to write simple JavaScript codes and add them to HTML.